Back to Developers
Get started with iOS
Okay iOS SDK Documentation | Secure Authentication for Developers
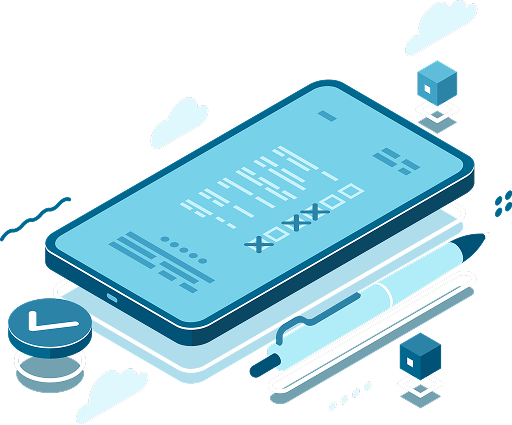
Our solution is based on server-side integration and SDK which has to be done on the mobile-side. It uses Apple push notification services to wake up the SDK when a transaction should be approved using SCA.
If you’d like to test out the Okay solution, we strongly recommend you to try out our application on play store or app store, which already has the Okay SDK integrated. You can also take a look at our guide, which explains how to Integrate the Okay app with your service. If you’d like to use our SDK with your iOS application, we also recommend you schedule a demo with us to help guide you through the integration process.
Kindly note, our SDK requires that your application can receive push notifications and is properly set up for the Okay Secure Server. If you have any questions or issues with the integration process, feel free to contact us at developer@okaythis.com.
Open Xcode then navigate to File -> Swift Packages -> Add Package Dependency. Enter depository URL as https://github.com/Okaythis/PSAiOS. Use “Next Major” as your default version rule.
To add the Okay SDK dependency to your application, add the following entry to your Podfile:
pod 'PSASDK', '~> 1.2.2'
Then run the pod install command in your terminal. You can find a sample application here to help guide you with the SDK installation.
- Open the project’s general tab.
- Add PSA, PSACommon and DefaultPsaUi frameworks to your embedded binaries section in Xcode.
Click the links below to download the required zipped frameworks.
zip with PSA framework
Please don't forget to import the framework in your application with import PSA.
The SDK is compatible with both devices and simulators, but multi-factor authentication is only available on real devices because of the push notification services requirement.
In order to use the SDK, you should enable push notifications for your app. In this example, we will use a test endpoint that will send push notifications after clicking the button.
The Okay service allows tenants to be able to configure the Okay service to use Firebase as the Push notification service provider for iOS.
In order to have the Okay service send push notification requests through Firebase to iOS devices, you have to select Firebase as your Notification Provider for iOS and you are also required to provide your Firebase cloud messaging server key on your tenant settings page which can be found here.
For more information on how to add Firebase to your iOS project, please see the guide at https://firebase.google.com/docs/ios/setup.
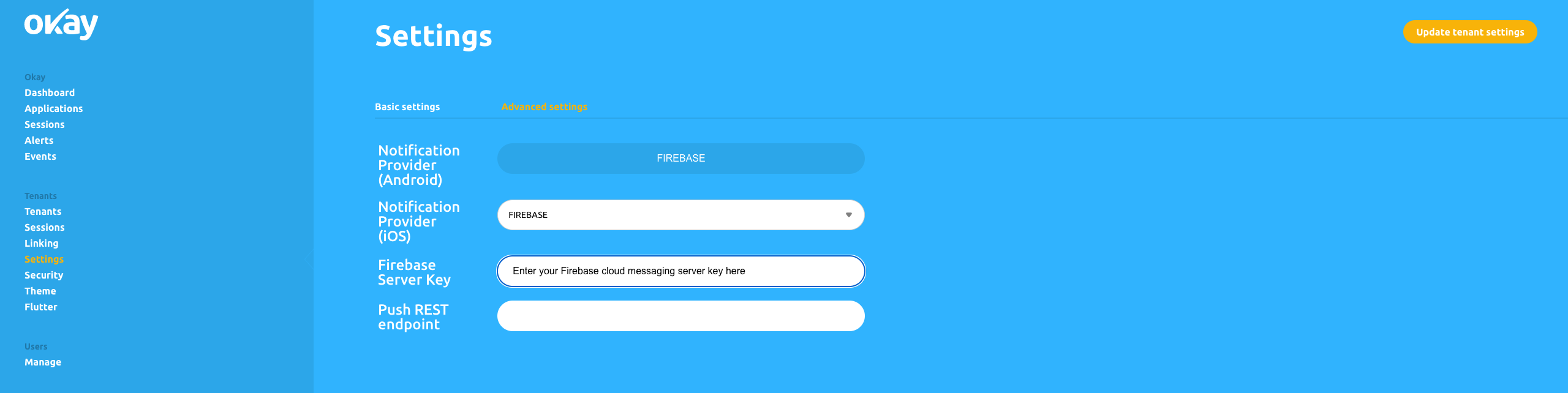
When you have got push notifications enabled and the device push notification token is received on the device, you can register it with Okay Secure SDK with the following method call:
PSA.updateDeviceToken(deviceToken: String!)
Now you’re ready for enrolling.
Use the following as your installationId and pubPssBase64 key respectively:
installationId: 9980
pubPssBase64: MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAxgyacF1NNWTA6rzCrtK60se9fVpTPe3HiDjHB7MybJvNdJZIgZbE9k3gQ6cdEYgTOSG823hkJCVHZrcf0/AK7G8Xf/rjhWxccOEXFTg4TQwmhbwys+sY/DmGR8nytlNVbha1DV/qOGcqAkmn9SrqW76KK+EdQFpbiOzw7RRWZuizwY3BqRfQRokr0UBJrJrizbT9ZxiVqGBwUDBQrSpsj3RUuoj90py1E88ExyaHui+jbXNITaPBUFJjbas5OOnSLVz6GrBPOD+x0HozAoYuBdoztPRxpjoNIYvgJ72wZ3kOAVPAFb48UROL7sqK2P/jwhdd02p/MDBZpMl/+BG+qQIDAQAB
if PSA.isReadyForEnrollment() {
PSA.startEnrollment(withHost: “https://demostand.okaythis.com”,
invisibly: true,
installationId: installationIdString,
resourceProvider: nil,
pubPssBase64: pubPssBase64String) { status in
// Handle enrollment status
}
}
Where:
- host: should be the link to Okay Secure Server address.
- Invisibly: is a parameter that describes whether the enrolment screen should be shown or not
- installationId: is used as an application identifier
- resourceProvider: object for passing additional data into the SDK
- pubPssBase64: is a key used for request headers encryption. installationId and
pubPssBase64: should be the same on both the app and the server. - status: parameter to be passed in closure describes enrolment status
If enrollment was completed successfully, then you’re ready to receive notifications.
To check if the app is already enrolled, use the following method:
PSA.isEnrolled() -> Bool
In order to be able to send authorisation requests to a user’s device using the Okay service, you will need to link that user to Okay. The Okay SDK provides the following method for linking operations:
PSA.linkTenant(withLinkingCode: linkingCode){ (linkingStatus, tenant) in
if(linkingStatus.rawValue == 1){
print("linking with \(String(describing: tenant.tenantId)) was successful")
} else{
print("linking failed")
}
}
See this guide for more information on how to generate linking codes. Once your device is linked you can now proceed to sending authorisation/authentication requests to a user’s device.
Okay sends authentication/authorisation session details via push notification to a user’s linked device.
In order to be able to start an authorisation session with the Okay SDK, you need to extract the sessionId parameter from the push notification that Okay sends to the linked device. The following sample code is an example of how you can retrieve the sessionId from the push notification.
let pss = notification.request.content.userInfo["pss"] as? [String: Any],
let data = pss["data"] as? [String: Any],
let sessionId = data["sessionId"] as? NSNumber
Now you can check if authorisation with Okay SDK is available in the application by calling the following method:
PSA.isReadyForAuthorization() -> Bool
The Okay SDK provides the startAuthorisation method which is used to start an authorisation session. In order to start an authorisation session with the Okay SDK you are required to pass in the sessionId to this method.
PSA.startAuthorization(with: PSATheme?,
sessionId: NSNumber?,
resourceProvider: ResourceProvider?,
loaderViewController: UIViewController?, completion: (Bool, PSASharedStatuses, TransactionInfo?) -> Void)
For example:
let sessionId = pushNotificationData["sessionId"]
PSA.startAuthorization(with: nil, sessionId: NSNumber(value: sessionId as! Int), resourceProvider: nil, loaderViewController: nil) { (isCancelled, status, _) in
if(!isCancelled && status.rawValue == 1){
print("Auth was successful")
}else{
print("Auth was not successful")
}
}
Where
- psaTheme: An object that allows customisation to colours and fonts on the authorisation screen
- sessionId: A numeric value that is parsed from the received push notification
- resourceProvider: - An object used to pass additional data into the SDK such as text to be displayed to the user during enrolment.
- loaderViewController: - UIViewController object which is shown as a loader screen before the authorisation screen when the SDK communicates with the Okay server
- isCancelled: closure parameter describes if authorisation was cancelled or confirmed
- status: This parameter describes the authorisation completion status
- transactionInfo: This is an object that contains the completed authorisation result
PSATheme describes colours and fonts on authorisation screen.
PSATheme is a class that describes the style of authorization screens.
@property (strong, readonly, nullable) UIImage *logo;
@property (copy, readonly, nullable) NSString *title;
- (instancetype)initWithTitle:(nullable NSString *)title;
- (void)setResponseDictionary:(nullable NSDictionary<NSString *, id> *)responseDictionary;
- (UIColor *)getColorForKey:(nullable NSString *)key;
@end
To change the authorisation screens style or appearance, you can set a dictionary with the following keys:
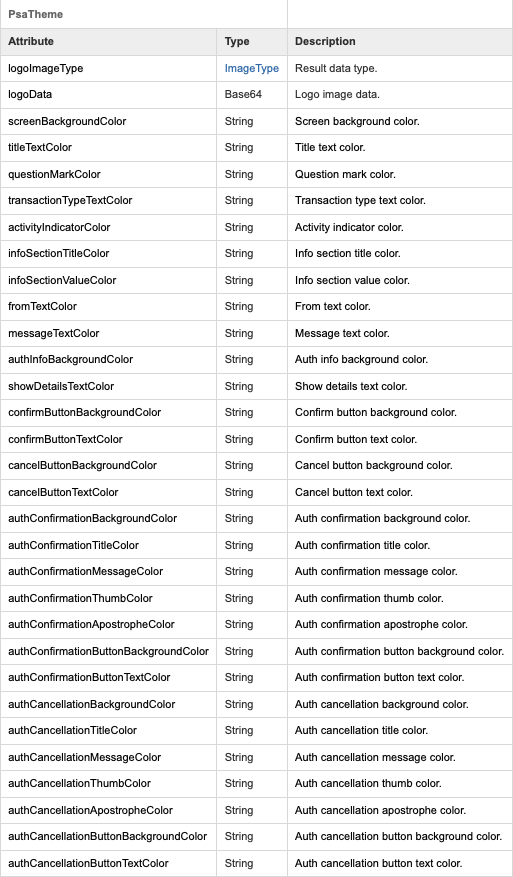
The following images below will help you understand which key you need.
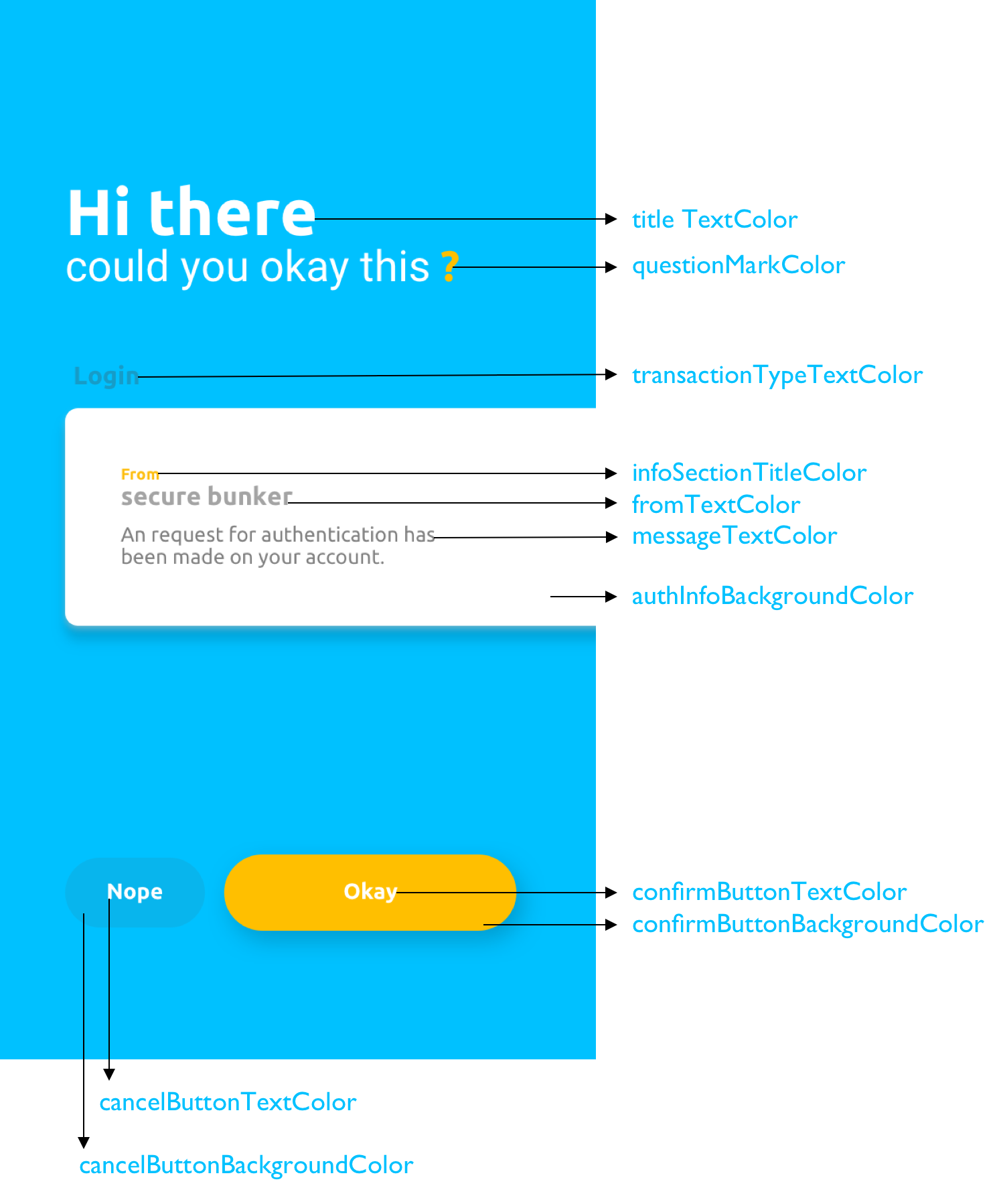
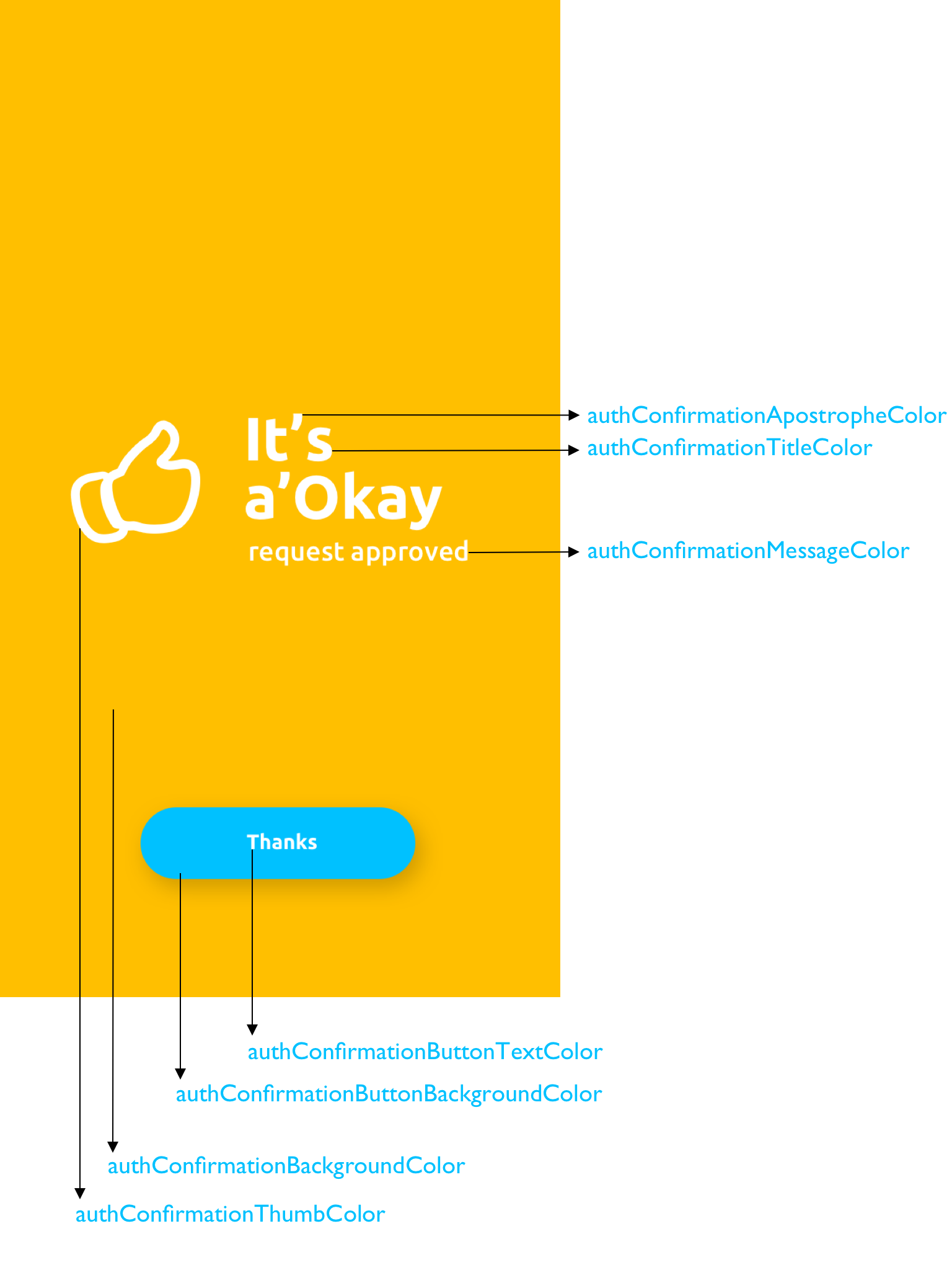
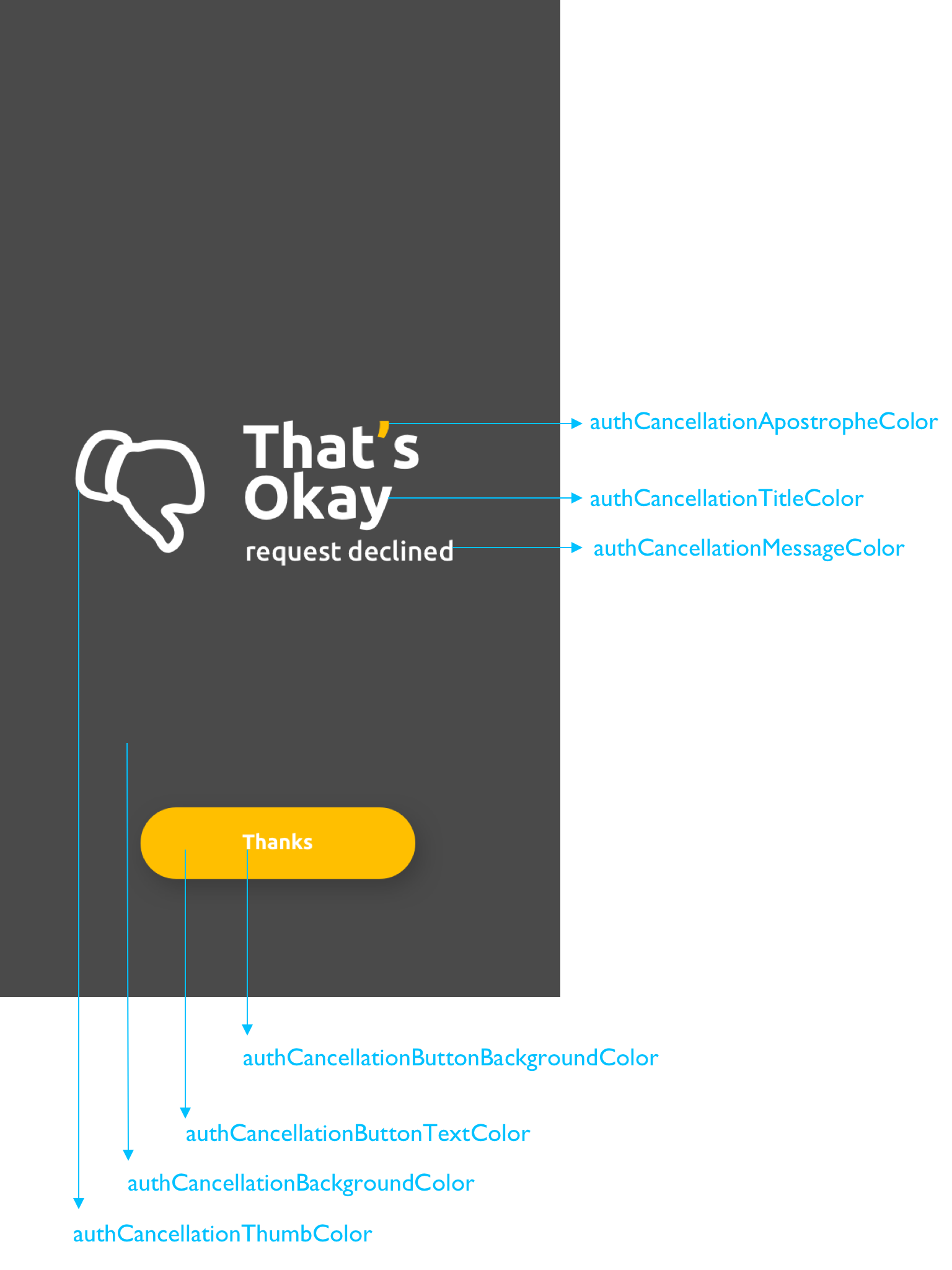
The Okay secure SDK allows the backend to retrieve information about a user’s device, such as:
- Push notification settings.
- Biometric sensor availability or Biometric identification capability on the user device.
When the user device information is requested, the app receives push notification containing session type with value 30. Session type can be extracted as shown in the sample code below:
func fetchSessionType(fromNotification notification: UNNotification) -> Int? {
guard let pss = notification.request.content.userInfo["pss"] as? [String: Any] else {
return nil
}
return pss[“type”] as? Int
}
After retrieving session type use the following method to send the device settings to the server:
PSA.sendDeviceLivelinessSettings(completion: (PSASharedStatuses) -> Void)
The passed completion handler receives the session completion result.
To use the Okay SDK, you need installationId and pubPss key. They are issued for every version.
iOS:
PSMP Version: 1.0.3
Installation: 9980
MIIBCgKCAQEAxgyacF1NNWTA6rzCrtK60se9fVpTPe3HiDjHB7MybJvNdJZIgZbE
9k3gQ6cdEYgTOSG823hkJCVHZrcf0/AK7G8Xf/rjhWxccOEXFTg4TQwmhbwys+sY
/DmGR8nytlNVbha1DV/qOGcqAkmn9SrqW76KK+EdQFpbiOzw7RRWZuizwY3BqRfQ
Rokr0UBJrJrizbT9ZxiVqGBwUDBQrSpsj3RUuoj90py1E88ExyaHui+jbXNITaPB
UFJjbas5OOnSLVz6GrBPOD+x0HozAoYuBdoztPRxpjoNIYvgJ72wZ3kOAVPAFb48
UROL7sqK2P/jwhdd02p/MDBZpMl/+BG+qQIDAQAB
-----END RSA PUBLIC KEY-----
MIIBCgKCAQEAxgyacF1NNWTA6rzCrtK60se9fVpTPe3HiDjHB7MybJvNdJZIgZbE
9k3gQ6cdEYgTOSG823hkJCVHZrcf0/AK7G8Xf/rjhWxccOEXFTg4TQwmhbwys+sY
/DmGR8nytlNVbha1DV/qOGcqAkmn9SrqW76KK+EdQFpbiOzw7RRWZuizwY3BqRfQ
Rokr0UBJrJrizbT9ZxiVqGBwUDBQrSpsj3RUuoj90py1E88ExyaHui+jbXNITaPB
UFJjbas5OOnSLVz6GrBPOD+x0HozAoYuBdoztPRxpjoNIYvgJ72wZ3kOAVPAFb48
UROL7sqK2P/jwhdd02p/MDBZpMl/+BG+qQIDAQAB
-----END RSA PUBLIC KEY-----