Back to Developers
React Native SDK documentation
Use our SDK for React Native apps
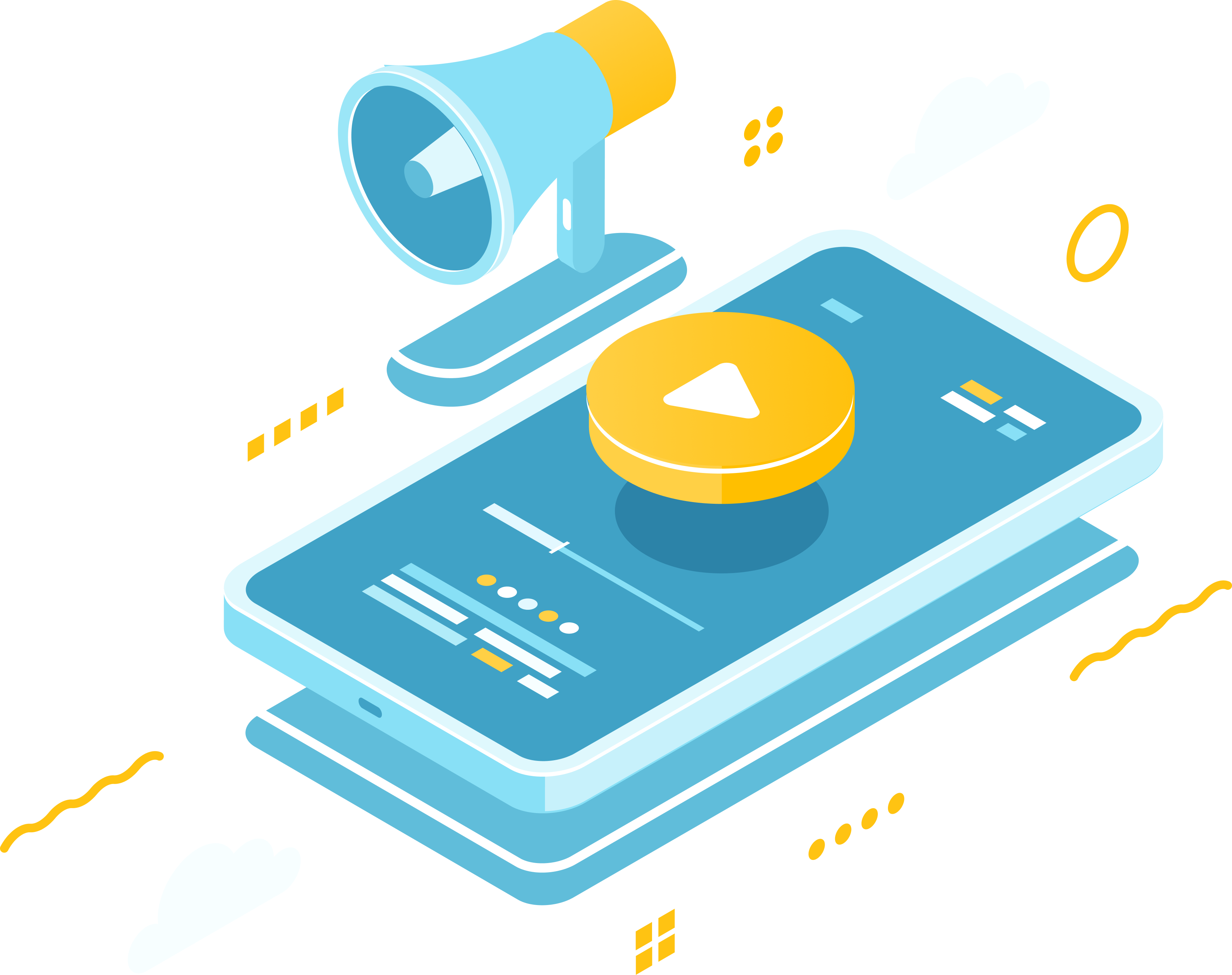
$ mkdir ~/project_dir/custom_modules
$ cp ~/Downloads/RNOkaySDK ~/project_dir/custom_modules
"react-native-okay-sdk": "file:custom_modules/RNOkaySDK"
Run the following command from your project root folder:
$ yarn install
Run the following command from your project root folder:
$ react-native link react-native-okay-sdk
Locate your project_dir/android/app/build.gradle file in your project workspace, then set your minSDKVersion in the build.gradle to API 21.
buildscript {
ext {
buildToolsVersion = "28.0.3"
minSdkVersion = 21 // use API 21
compileSdkVersion = 30
targetSdkVersion = 30
ndkVersion = "21.4.7075529"
}
}
Add the Okay maven repository to your Android project_dir/android/build.gradle file:
allprojects {
repositories {
mavenLocal()
google()
jcenter()
maven {
// All of React Native (JS, Obj-C sources, Android binaries) is installed from npm
url "$rootDir/../node_modules/react-native/android"
}
// Begin: Add This
maven {
url "https://gitlab.okaythis.com/api/v4/projects/15/packages/maven"
name "GitLab"
}
// End:
}
}
Locate your project_dir/android/src/main/AndroidManifest.xml file, then add these Android permissions to the file:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.READ_PHONE_STATE"/>
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
Add the following to your Android project_dir/android/app/build.gradle file:
android {
compileSdkVersion rootProject.ext.compileSdkVersion
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
// Begin Add DataBinding
dataBinding {
enabled = true
}
// End
defaultConfig {
...
multiDexEnabled true // Add this line
}
...
}
The Okay service uses Firebase push notification service to deliver 2FA push notifications to your app. Please see the following link for further instructions on how to install Firebase for React Native https://rnfirebase.io/.
Please visit this link to enable Push Notification for iOS devices when using React Native: https://facebook.github.io/react-native/docs/pushnotificationios
- initOkay(okayServerAdress: string): Promise (Android Only)
- updateDeviceToken(token: string): void (iOS only)
- isEnrolled(): boolean
- startEnrollment(enrollData: any): Promise;
- linkTenant(code: string, spaStorageData: any): Promise;
- unlinkTenant(id: number | string, spaStorageData: any): Promise;
- isReadyForAuthorization(): Promise;
- startAuthorization(spaAuthData: any): Promise;
We will need to call the initOkay(object) on the SDK to properly initialize the Okay SDK. For example we pass in 'https://demostand.okaythis.com' as our Okay server endpoint.
OkaySdk.initOkay({
initData: {
okayUrlEndpoint: 'https://demostand.okaythis.com',
},
})
For Okay to work correctly, you are required to prompt the user to grant the notification permissions on iOS.
import messaging from '@react-native-firebase/messaging';
// Snippet of permission request using Firebase SDK
const authStatus = await messaging().requestPermission();
console.log('status: ', authStatus);
const enabled =
authStatus === messaging.AuthorizationStatus.AUTHORIZED ||
authStatus === messaging.AuthorizationStatus.PROVISIONAL;
if (enabled) {
console.log('Authorization status:', authStatus);
messaging().getToken().then(token => {
console.log('token: ', token);
OkaySdk.updateDeviceToken(token || '');
})
}
We will need to update Okay SDK with the push notification token generated for iOS devices:
// We can update iOS PNS token in this lifecycle method here
// If you are using APNS your code will look similar to the following line
PushNotificationIOS.addEventListener('register', token => {
RNOkaySdk.updateDeviceToken(token);
});
// If you are using Firebase your code will look similar to this
messaging().getToken().then(token => {
console.log('token: ', token);
OkaySdk.updateDeviceToken(token || '');
})
If the required permissiosn have been granted on the device, we can now proceed to enrolling the user. Okay SDK provides the startEnrollment(enrollData: any) method which takes a Json with "SpaEnrollData" as key.
messaging().getToken().then(token => {
RNOkaySdk.startEnrollment({
SpaEnrollData: {
host: "https://demostand.okaythis.com/", // Okay server address
appPns: token,
pubPss: pubPssBase64,
installationId: "9990",
pageTheme: {
// Page Theme customization, if you don't want customization: pageTheme: null.
actionBarTitle: "YOUR_ACTION_BAR_TITLE",
actionBarBackgroundColor: "#ffffff",
actionBarTextColor: "#ffffff",
buttonTextColor: "#ffffff",
}
}
}).then(response => console.log(response));
})
SpaEnrollData contains several keys that are required for a secure communication with Okay servers.
"appPns": This is your push notification token from Firebase(or Firebase registration token for iOS devices if you are using Firebase for push notification on iOS) or APNS token if you are using APNS. This allows us to send 2FA notifications to your apps.
"installationId": The installationId is a unique value that identifies unique installation keys for the Okay SDK in an app. For testing purposes we ask our users to use this value 9990 as their installationId
"pubPss": This is a public key we provide to applications that use our SDK for secure communication with the Okay server. For testing purposes we ask our users to use the value below as their "pubPss" key.
const pubPssBase64 = 'MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAxgyacF1NNWTA6rzCrtK60se9fVpTPe3HiDjHB7MybJvNdJZIgZbE9k3gQ6cdEYgTOSG823hkJCVHZrcf0/AK7G8Xf/rjhWxccOEXFTg4TQwmhbwys+sY/DmGR8nytlNVbha1DV/qOGcqAkmn9SrqW76KK+EdQFpbiOzw7RRWZuizwY3BqRfQRokr0UBJrJrizbT9ZxiVqGBwUDBQrSpsj3RUuoj90py1E88ExyaHui+jbXNITaPBUFJjbas5OOnSLVz6GrBPOD+x0HozAoYuBdoztPRxpjoNIYvgJ72wZ3kOAVPAFb48UROL7sqK2P/jwhdd02p/MDBZpMl/+BG+qQIDAQAB'
"pageTheme": This is a JSON object that allows you to customize the colors for our enrollment and authorization screens to suit your product branding. Click here to see all valid color properties
The linkTenant(linkingCode, SpaStorage) method links a user of your application with an existing tenant on the Okay secure server. When you make a linking request to the Okay server, it returns a linkingCode as part of its response (For more information on how to send a linking request please see this documatation). The linking code can be passed directly to this method after a successful linking request to the Okay Service.
The externalId can be retrieved from the RNOkaySdk.startEnrollment(...).then( externalId => ...) method, if the method was called and executed successfully.
messaging().getToken().then(token => {
RNOkaySdk.linkTenant(
linkingCode,
{
SpaStorage: {
appPns: token,
pubPss: pubPssBase64,
externalId: 'YOUR_EXTERNAL_ID',
installationId: "9990",
enrollmentId: null
}
})
})
If a user was successfully linked to a tenant and you now wish to unlink that user from your tenant on the Okay secure server, you can use the unlinkTenant(tenantId, SpaStorage) method to do this as shown below.
messaging().getToken().then(token => {
RNOkaySdk.unlinkTenant(
tenantId,
{
SpaStorage: {
appPns: token,
pubPss: pubPssBase64,
externalId: 'YOUR_EXTERNAL_ID',
installationId: "9990",
enrollmentId: null
}
})
})
When there is a transaction that needs to be authorized by your application, Okay sends a push notification to your mobile app with the transaction details needed to complete that request. The body of the push notification has the following fields as it payload:
{
"tenantId": <int>,
"sessionId": <int>,
...
}
When the push notification is received on the client side, you should retrieve the sessionId from the push notification body. Pass in the sessionId value to RNOkaySdk.authorization(SpaAuthorizationData) method directly as shown in the code snippet below:
messaging().onMessage(async message => {
let data = JSON.parse(message.data.data);
let response = await OkaySdk.startAuthorization({
SpaAuthorizationData: {
sessionId: data.sessionId,
appPns: deviceToken,
pageTheme: null,
},
});
});